Advertisement
Game Manual
Scripting
1 What is a script?
A script is a group of commands that allow you to achieve a task. Scripts can be used for:• Improve control flexibility (e.g., multiple weapon binds)
• Execute multiple functions with one keystroke (e.g., demo recording)
• Facilitate config customization for multiple mods (e.g., Urban Terror)
After reading through this basic explanation of script writing you should be comfortable with writing your own basic scripts. Visit The Bind:Arena to see some examples of some really cool scripts and Commander Keen's Quake 3 Arena Console Page for all the different console commands and variables you might need while writing your scripts and Upset Chaps Quake3 & Team Arena Guide for additional information.
2 Script Organization
Scripts have to be saved in a separate .cfg file from your q3config.cfg. The reason is that Quake III overwrites the q3config file every time you start a game or change settings and therefore will delete your scripts and comments.I put all of my scripts into an autoexec.cfg. If you don't know how to put one together, visit the Tweaking Your Controls:Making an Autoexec section for a quick lesson. (ADD THIS LINK & SECTION)
As you write scripts, use // comments to help annotate what your script does. It will help better organize scripts in your configuration and if you want to give your scripts to other people, it makes it pretty clear what it does. Quake III will not execute anything that follows a pair of double-backslashes (//).
Example of (//) double-backslahses:
//Function Keys
bind F1 "ui_selectteam" //Displays Team Select Interface
bind F2 "ui_selectgear" //Displays Weapon & Gear Interface
bind F3 "ui_radio" //Displays radio menu
bind F3 "toggle cg_drawFPS" //Displays Frames per Second
bind F4 "toggle cg_drawTimer" //Displays timer
bind F5 "toggle cg_thirdperson" //Shows model in third person view
bind F6 "toggle cg_drawhands" //Shows hands & weapon
bind F11 "screenshot" //Takes screenshot
You can also use the Echo command (more on this later) to give yourself in-game messages when a script has been activated. We'll discuss this later, but you can go to some of the examples section and look at the zoom toggle and the demo recording script to see what in-game messages I give myself when the script is activated.
3 Basic Binds
Binding a key to perform a single action is the easiest place to start. We'll start by binding the 'X' key to select the SPAS12 (weapon 4). The syntax is:bind key "command"
Since we want to bind 'X' to the SPAS12, we will substitute 'X' for key and 'weapon 7' for command. This will give us:
bind x "weapon 7" //Select SPAS12
That was simple enough, let's move on.
4 Multiple Binds
We can also bind a key to execute multiple commands. Remember that Quake III executes commands in the order you've specified so we have to be careful. For example, we are going to bind a key to select the best choice between the SPAS12 (weapon 4) and the G36 (weapon 9). The syntax is:bind key "command 1; command 2" //Comments go here
You have to separate the commands with a semicolon (;) so that Quake recognizes where each command starts and stops. We're going to use the 'X' key again for key, weapon 4 for command 1, and weapon 9 for command 2. This should give us:
bind X "weapon 4; weapon 9" //Select best weapon Spas12 and G36
When you hit 'X', the bind selects the first weapon (e.g., weapon 4) then immediately selects the second weapon (e.g., weapon 9). In effect, if you have both weapons in your inventory, it selects the last one in the sequence.
If you only have one of the weapons, it will only find and therefore activate that weapon. This is why we want to make sure we write weapon 4 first since given the choice between the two, we always want to end up with the G36.
You can string a whole bunch of commands together to be executed all at once. This bind for example, will take a remove all the clutter from your screen (e.g., icons, guns, etc), take a screenshot, and then return your HUD to the way it was :
bind F11 "cg_draw2d 0;cg_drawhands 0; wait; wait; wait; screenshot; toggle cg_draw2d; toggle cg_drawhands"
Note: Try this bind see if it works
5 Recursive Scripts
Instead of only selecting the best weapon, what if we want to toggle back and forth between two weapons like the UMP45 and the GM4 or make it possible to zoom to 3x or 6x magnification? This requires a script, which we will call the recursive script. By recursive, it means that you can cycle through the script an infinite number of times. The intent is to execute one string of commands when you hit the bound key, then re-bind the key to execute new string of commands the next time you use the key. The process is:Name your script and add a short description of what it does:Add your name and description after a comment (// - double-backslash) so that Quake III doesn't try to recognize it as a command. Assigning a good descriptive name will help you find your script later, and if you decide to share it with others, helps them understand what they're getting (e.g. // 3x - 6x zoom toggle).
Write commands for each 'step' in the script:
set scriptline(n) "command; set nextscriptline vstr scriptline(n); echo comment"
The 'set' command tells Quake that you are defining a line of commands that will be grouped and executed under the name scriptline.
• scriptline(n) is the name of one iteration or step in your script. If you have a large number of steps, start numbering with 1. If you have 4 steps, then the highest number should be 4. For scripts with a small number of steps, you can use simple names, just make sure that you don't use the same name twice.
• command is the command or set of commands you want to execute with the script. Multiple commands should be separated by a semicolon (;) like we learned in the Multiple Binds section.
• set nextscriptline tells the script to assign nextscriptline to execute the string of commands defined in scriptline(n).
• vstr scriptline(n) is the name of the next alias in the sequence to be activated. Look at the weapon scripts. In the first iteration, I want to choose the G36 over the SPAS12, but in the second, I want to reverse the sequence. In your last iteration, make n=1 to start all over from the beginning.This will let your key cycle through all of the combinations you've written, then start all over from the beginning. You don't have to use a numerical sequence, but it might make it easier to keep track of. In any case, pick whatever you're comfortable with.
• echo comment Any text that follows the echo command will be shown to you as an in-game message. I find this useful for reminding me what my script just did.
Here's an example for a 3x - 6x zoom toggle:
set zoomtoggle1 "cg_zoomfov 15; sensitivity 25; set nextzoomtoggle vstr zoomtoggle2; echo 6x magnification"
set zoomtoggle2 "cg_zoomfov 30; sensitivity 17; set nextzoomtoggle vstr zoomtoggle1; echo 3x magnification"
Assign dynamic variable to first 'step' in sequence: After you have finished writing the basic set of commands, use set nextscriptline vstr scriptline(n)
This tells the computer that nextscriptline should start by executing scriptline(n). Notice however, that every time you execute your script, the value attached to nextscriptline changes. Here's an example for a 3x - 6x zoom toggle: set nextzoomtoggle "vstr zoomtoggle1"
Bind key to execute dynamic variable: The last step is to bind a key to execute your new script. We learned the syntax in the first step, Simple Binds. The final line in the zoom toggle looks like: Here's an example for a 3x - 6x zoom toggle:
bind b "vstr nextzoomtoggle"
All this does is bind the key to execute the string of commands associated with nextscriptline. Here's what it looks like when it's all put together:
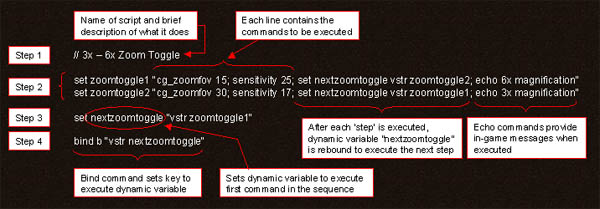
Try this out and play around a little. You'll be writing your own scripts in no time.
6 Toggles
Any command that can be set to either "on" or "off", can be set up on a toggle. For example, you can set Always Run to either on (1) or off (0), therefore I can create a toggle that lets me switch between always on or always off. This has a couple of advantages:• In the case of the +speed (run) command, I don't have to hold down my SHIFT key to run or walk. If I want to sneak up on someone, I can hit the toggle to walk, and then hit it again when I want to resume running.
• This set up conserves keys. Instead of one button set to enable always run and another to disable it, I can consolidate everything to one key.
The syntax is:
bind key "toggle command"
Here are a couple of examples:
bind SHIFT "toggle cl_run"
bind F3 "toggle cg_drawFPS" //Displays Frames per Second
bind F4 "toggle cg_drawTimer" //Displays timer
bind F5 "toggle cg_thirdperson" //Shows model in third person view
bind F6 "toggle cg_drawhands" //Shows hands & weapon
Look through your configuration for variables that take either a 0 or 1 value. Those are the commands that can be set up on a toggle.
7 Executables
This command can be used to clean up the autoexec.cfg. Basically, this is a file containing each script. For example my favorite servers, one with communications binds and scripts, and a third for demo recording/handling binds.Having them in a separate file lets me share them with teammates, team comm binds for example, or pass along my favorite servers without having to send the entire autoexec.
The other reason for this is config organization. If your configuration to too large, Quake III will have problems executing the whole thing. Seperate configs set up as executables enable you to reduce the overall size of your primary autoexec. The syntax is:
exec filename.cfg
Here are a couple of examples that could be used in an autoexec.cfg:
exec comm.cfg
exec serverlist.cfg
exec demo.cfg
8 Examples
Here are just a few example of scripts that can be used in your in your config.// Toggle Sprint, Run, Walk Bind
set mz_00 "set mz_f vstr mz_01; -button8; +speed; ut_echo SPEED: WALKING"
set mz_01 "set mz_f vstr mz_02; -button8; -speed; ut_echo SPEED: RUNNING"
set mz_02 "set mz_f vstr mz_00; +button8; -speed; ut_echo SPEED: SPRINTING"
set mz_f "vstr mz_01" //sets run as default
bind SHIFT "vstr mz_f" //bind for changing speed
// Toggle Crouch Bind
set sit "+movedown; set crouch vstr stand"
set stand "-movedown; set crouch vstr sit"
set crouch "vstr stand" //set default
bind c "vstr crouch"
9 Why does my script not work?
There are two possible errors. One of them happens when your script is too big. All scripts should stay under 16KB in size, to solve this problem break the script or scripts into smaller files which you can then execute by adding the following lines to your autoexec.cfg (where FileName is the name of your files):exec FileName_1.cfg
exec FileName_2.cfg
If you have a lot of such sub scripts we advise you create a folder in which to store them, to keep your game directory fairly tidy. This can be done by creating a new folder with the name of your choice (we will name it "cfg" in our exemple) in your q3ut4 folder. Now place all your sub scripts into this folder, the autoexec.cfg should NOT be placed in this folder. Now add the following lines to your autoexec.cfg:
exec cfgFileName_1.cfg (where cfg is your folder and FileName is the name of your sub-script)
exec cfgFileName_2.cfg
Another possible error is triggered when you have too many cvar strings active (i.e too many scripts) this error is usually accompanied by an error message similar to "insert text overflow" the only way to solve this issue is to start hacking away at what you really don't need in your scripts. There are some ways around this with some creative scripting but that is beyond the scope of this manual.
10 Where can I find script information?
If you would like to learn more about modifying your config file, we recommend:Most of these sites are no longer updated, but the information contained within can still be applied to Urban Terror. We are still on the look out for Zex Suik, curator of UT Scripts. If we can find him and retreive his files we will include those on the web site in the future.
By FrozenSand - Wednesday, 28 October 2009 - viewed by 6361 members and 87608 visitors
Advertisement